A Text
widget in Flutter is a very commonly used widget which is used to display a block of text on the screen. The text that we want to display using the Text
widget can either be static or it can be dynamic in nature.
So if you have some text in the Text
widget and you want to change it dynamically such as on a button click, then you can follow the below steps:
- First, Create a custom stateful widget class by extending the
StatefulWidget
class. - Declare a variable eg. dynamicText inside the State class of the stateful widget which will hold the value of the dynamically changing text.
- Put the
dynamicText
variable inside the target Text widget eg. Text(“$dynamicText”) - Create a custom function such as updateText() inside the State class. Inside this function, call the setState() method and inside the callback of the setState() method put the logic to change the text dynamically.
- Call the updateText() method inside the
onPressed
callback of the ElevatedButton(or any other button).
Here is a full Flutter example that changes the text of the Text widget on the button click:
import 'package:flutter/material.dart'; void main() { runApp(MyApp()); } class MyApp extends StatelessWidget { MyApp({super.key}); // App and app bar title static const String _title = 'Change Text Dynamically'; @override Widget build(BuildContext context) { return MaterialApp( title: _title, home: Scaffold( appBar: AppBar(title: const Text(_title)), body: const Center(child: TextChanger()), ), ); } } class TextChanger extends StatefulWidget { const TextChanger({super.key}); @override State<TextChanger> createState() => _TextChangerState(); } class _TextChangerState extends State<TextChanger> { // Declare the variable String dynamicText = 'Initial Text'; updateText() { setState(() { dynamicText = 'This is new text value'; // Replace with your logic }); } @override Widget build(BuildContext context) { return Column( children: [ Text( '$dynamicText', // Dynamic text style: const TextStyle(fontSize: 28), ), ElevatedButton( child: const Text('Change Text'), onPressed: () => updateText(), // Call the method ), ], ); } }
Output:
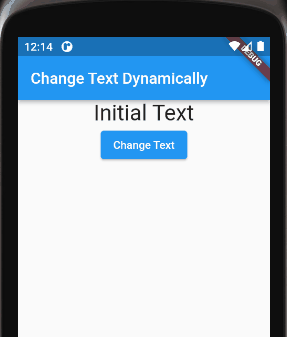
Code Explanation:
In the above example, we have explicitly called the setState() method inside the updateText() method which is invoked as soon as you click the button.
When the setState() method is called, Flutter rebuilds the widget tree by calling the build() method. The build() method then uses the updated value of the dynamicText
field and hence the text of the Text
widget changes instantly.
Conclusion
In this article, we learned how we can change the Text
widget’s text on button click.
In summary, if you want to change the Text
widget’s text on button click, you can create a stateful widget class by extending to the State
class of Flutter and then call its setState() method on the button press.
When the setState() method is called, the widget tree rebuilds, and the updated text reflects in the Text widget.
Thanks for reading.