The easiest way to scroll to the bottom of an element in JavaScript is to use the scrollTop
and the scrollHeight
properties of the DOM in combination.
The scrollTop
property specifies the distance in pixels from the top of an element to the position to where its content should be scrolled vertically. Whereas, the scrollHeight
property specifies the total height of an element in pixels i.e. including the visible and non-visible(when there are scrollbars) content.
So, if we want to scroll to the bottom of an element, we have to first find out the total height(including non-visible content’s height) of the element and then we can simply set it to the scrollTop
property.
Along with these two properties, we also have to set a fixed height for the element and then use the overflow property to show the vertical scrollbar.
Let me show you this with an example.
Let’s say we have a div element which has a fixed height of 200px. We have added a little more content inside of it so that it can flow out of its boundaries and set the overflow
property to auto
which will add scrollbars to the div to keep the content within its boundaries.
We have also added a button that we will use to scroll to the bottom of the div.
Here is the HTML:
<!-- Div whose content will be scrolled --> <div id="myDiv"> <p>This is some dummy content.</p> <p>This is some dummy content.</p> <p>This is some dummy content.</p> <p>This is some dummy content.</p> <p>This is some dummy content.</p> <p>This is some dummy content.</p> <p>This is some dummy content.</p> <p>This is some dummy content.</p> <p>This is some dummy content.</p> <p>This is some dummy content.</p> <p>This is some dummy content.</p> <p>This is some dummy content.</p> <p>This is some dummy content.</p> <p>This is some dummy content.</p> <p>This is some dummy content.</p> <p>This is some dummy content.</p> <p>This is some dummy content.</p> </div> <!--Button to initiate scroll to the bottom --> <button id="myBtn">Scroll to Bottom</button>
Now, along with the height
and overflow
properties, we also have to set the scroll-behavior property to smooth
. The scroll-behavior
property specifies the scroll behavior of an element and when we set it to smooth
, the scroll happens smoothly.
So, add the below code in the CSS:
div{ height: 200px; overflow: auto; scroll-behavior: smooth; border: 2px solid red; }
Next, add the below JS code:
let div = document.getElementById('myDiv'); let btn = document.getElementById('myBtn'); // Fires on button click btn.addEventListener('click', function(){ // Scroll to the bottom of the div div.scrollTop = div.scrollHeight; });
Below is the output of the above code:

As you can see from the above output, as soon as we click the button, it scrolls us smoothly to the bottom of the div element.
Scroll to the Bottom of an Element using the scroll() Method
You can also use the scroll()
method of the Element
interface to scroll to the bottom of an element.
The scroll()
method lets you scroll to a specific position inside an element based on the parameters passed to it. These parameters can be the X, Y Coordinates, or the top and left positions in pixels.
In our case, we want to scroll smoothly to the bottom of the element, so we will have to pass the distance from the top of the element in pixels.
Let’s take the same example that we discussed in the previous example and try to achieve the same behavior using the element.scroll()
method.
let div = document.getElementById('myDiv'); let btn = document.getElementById('myBtn'); // Fires on button click btn.addEventListener('click', function(){ // Scroll to the bottom of the div div.scroll({ top: div.scrollHeight, behavior:'smooth', }); });
This will give you the following output:
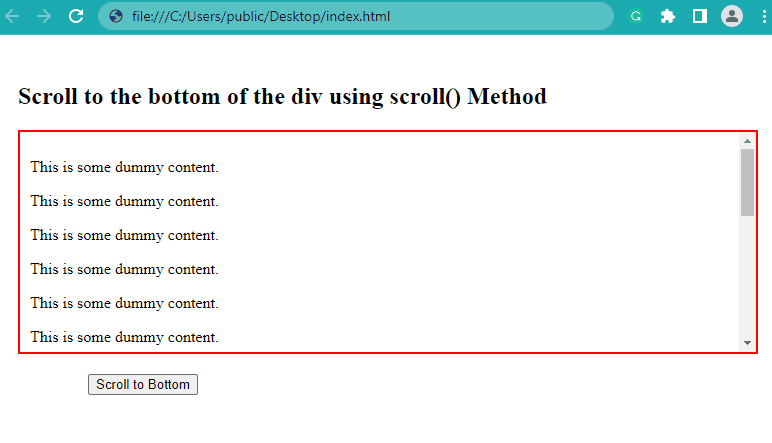
Conclusion
In this article, we discussed two ways to scroll to the bottom of an element using JavaScript.
To scroll to the bottom of an element, you can use the scrollTop
and scrollHeight
properties in combination. The scrollTop
property specifies the position of the vertical scroll in pixels whereas the scrollHeight
property specifies the total height of an element including the height of the hidden(scrollable) content.
An alternative approach could be to use the scroll()
method of the Element
interface.
Thanks for reading.