Checkboxes are widely used elements in modern applications. You can use them inside forms, tables, dropdowns, etc. to allow the user to select one or multiple options from a group of options.
When you use these checkboxes inside a form, you might sometimes need to disable them based on some condition, for eg. the user hasn’t typed the correct value inside an input field, or hasn’t selected the right value from the dropdown etc.
So, if you want to disable a checkbox in Angular, you have to bind the disabled
attribute of the checkbox to a boolean variable in your component.ts file.
After binding, if the value of that boolean variable is true, the checkbox will become disabled i.e. no pointer events will be allowed. On the other hand, if the value of that boolean variable is false, the checkbox will automatically become enabled.
Let’s try to understand this with an example.
Suppose we have a checkbox and two buttons in our component’s template file. These two buttons will make the checkbox disabled or enabled based on the click event.
To accomplish that we have added two click event handler functions makeDisable()
& makeEnable()
to the buttons which will be triggered every time you click the buttons and will make the checkbox disabled or enabled.
<div> Accept: <input type="checkbox" [disabled]="isDisabled" > <button (click)="makeDisable()">Disable</button> <button (click)="makeEnable()">Enable</button> </div>
Now, inside our ts file, we have to define the makeDisable() and makeEnable() functions, which will make the checkbox disabled and enabled respectively on the button click.
Inside the makeDisable() function, we will set the isDisabled
variable’s value to true which will make the checkbox disabled. On the other hand, we will set the isDisabled
to false inside the makeEnable() function.
import { Component } from '@angular/core'; @Component({ selector: 'app-home', templateUrl: './home.component.html', styleUrls: ['./home.component.css'] }) export class HomeComponent { // Keep the checkbox enabled by default isDisabled: boolean = false; constructor() { } ngOnInit(): void { } makeDisable(){ // Make the checkbox disabled this.isDisabled = true; } makeEnable(){ // Make the checkbox enabled this.isDisabled = false; } }
Below is the outcome of the above program:
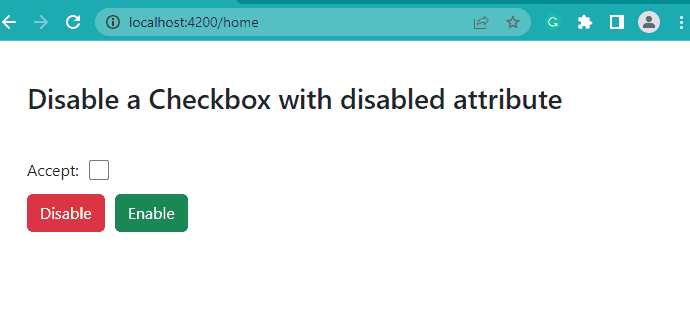
As you can see from the above output, the checkbox becomes disabled as soon as you click the first button, whereas, the checkbox becomes enabled as soon as you click the second button.
Disable a Checkbox Inside a Reactive Form
The above method that we discussed is mostly used to disable form elements within template-driven forms in Angular.
But, if you are working with Angular’s reactive forms, there is a much simpler way to do this.
To disable a checkbox in a reactive form, you can use the disable()
method of the FormControl
class. When you call the disable()
method on form elements such as inputs, radio buttons, checkboxes, etc. it makes them non-interactive.
For example, suppose we have a reactive form named userForm which has several form elements including a checkbox with a form control named ‘myCheckbox’:
userForm: FormGroup; constructor(private formBuilder: FormBuilder) { // Create the form group this.userForm = this.formBuilder.group({ fullName: ['', Validators.required], email: ['', Validators.required], password: ['', Validators.required], myCheckbox: [false] }); }
To disable the checkbox with form control named 'myCheckbox'
, we can call the disable()
method on it as follows:
// Make the form control named 'myCheckbox' disabled this.userForm.get('myCheckbox')?.disable();
Similarly, if you want to enable the checkbox(or any other form element), you can use the enable
() method of the FormControl
class.
See the following example:
// Make the form control named 'myCheckbox' enabled this.userForm.get('myCheckbox')?.enable();
Conclusion
In this article, we learned how we can make a checkbox disabled or enabled in Angular.
One way to make a checkbox disabled in Angular is to use the disabled
attribute on the checkbox. The disabled
attribute is a boolean attribute. If its value is set to true, the checkbox will become disabled otherwise it will become enabled.
If you are using the checkbox inside a reactive form, you can use the disable()
and enable()
methods to make the checkbox disabled or enabled.
Thanks for reading.